Electronでアプリを開発しようと思ったときに、最初にやるのは、シンプルな構成でまず動かしてみることですよね。
今回は、そのやり方を解説します。
以前の記事では、electron公式のサイトのアナウンスされている、サンプルアプリの動かし方と、デバッグ環境の用意の仕方を解説したので、参考にしてください。
Node.jsの用意
最初にnode.jsをインストールします。
electronはnode.jsで動くからです。
nodeのインストール方法はここを参照してください。
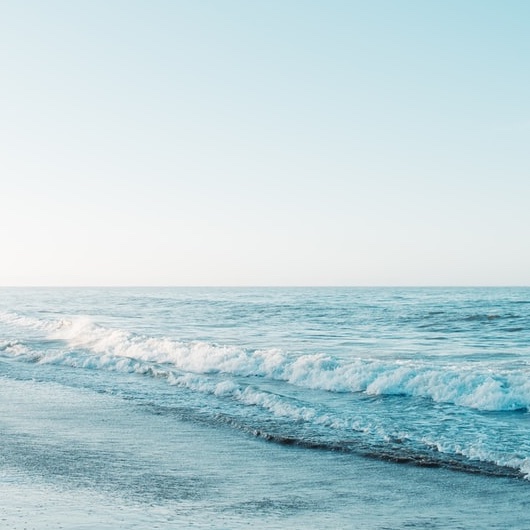
electronのインストール
node.jsをインストールしたら、Node.jsのコマンドプロンプトを立ち上げます(windowsの場合)。
その後、アプリを開発したいディレクトリを作成し、移動します。electron01というディレクトリ配下で開発していくとしたら以下のコマンドを打ちます。
mkdir electron01
cd electron01
で、次のコマンドで、空の新しいnodeプロジェクトを作成・初期化します。
すると、プロジェクトフォルダの直下に「package.json」が出来上がります。
npm init
さて、空のnodeプロジェクトができたので、electronのライブラリを使えるようにしましょう。
npm install electron -g
と打つと、node環境全体としてelectronのライブラリがインストールされます。
全体で使える反面、プロジェクトごとにバージョンの異なるelectronで開発できません。
新しいelectronのバージョンで開発したい場合もありえますので、プロジェクトのローカルにelectronをインストールしましょう。
ローカルにインストールするには、以下のコマンドを使います。
npm install --save-dev electron
シンプルなアプリの作成
最小構成は以下のような感じなので、main.jsとindex.htmlを作ってみよう。
your-app/
├── package.json
├── main.js
└── index.html
const { app, BrowserWindow } = require('electron')
function createWindow () {
// Create the browser window.
const win = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true
}
})
// and load the index.html of the app.
win.loadFile('index.html')
// Open the DevTools.
win.webContents.openDevTools()
}
app.whenReady().then(createWindow)
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') {
app.quit()
}
})
app.on('activate', () => {
if (BrowserWindow.getAllWindows().length === 0) {
createWindow()
}
})
<title>Hello World!</title>
<!-- https://electronjs.org/docs/tutorial/security#csp-meta-tag -->
<h1>Hello World!</h1>
We are using node document.write(process.versions.node),
Chrome document.write(process.versions.chrome),
and Electron document.write(process.versions.electron).
最初にデフォルトで出来たpackage.jsonを変更します。
変更箇所は、
“main”: “main.js”,
の部分と、
“start”: “electron .”
の部分を追加することです。
{
"name": "electron01",
"version": "1.0.0",
"description": "",
"main": "main.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "electron ."
},
"author": "",
"license": "ISC",
"devDependencies": {
"electron": "^9.1.0"
}
}
で、
npm start
をコマンドプロンプトで打つと、アプリが起動します。